Async-Await Magic: How it Simplifies Asynchronous Programming
Asynchronous programming can be a daunting task, with callbacks, promises, and nested code quickly leading to “callback hell.” But fear not, for there is a magic spell that can tame the asynchronous beast: async/await.
Async-Await Magic: What is Async/Await?
Async/await, introduced in ECMAScript 2017, is a set of keywords providing a cleaner and more intuitive way to write asynchronous code. It allows you to write asynchronous code in a synchronous-like style, making it easier to read and understand.
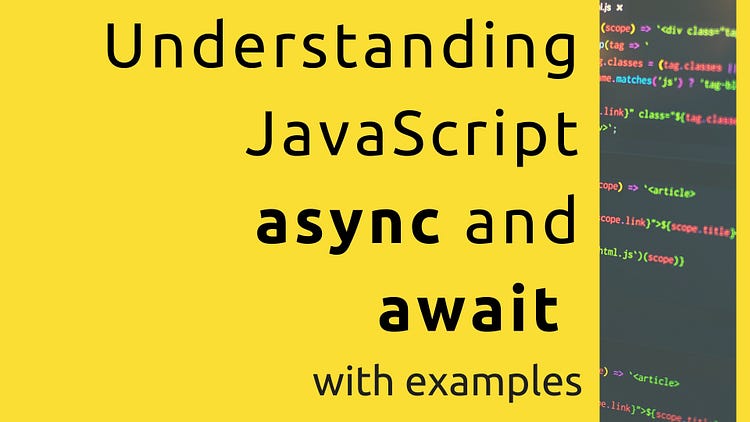
Async-Await Magic: How Does it Work?
- Async Functions: Start by defining an async function, capable of performing asynchronous operations.
- Await Keyword: Inside the async function, use the await keyword before any asynchronous operation, such as fetching data from an API or reading a file.
- Suspension and Resumption: When the await keyword is encountered, the execution of the async function is suspended until the asynchronous operation is complete. Meanwhile, the JavaScript runtime can execute other code.
- Resuming Execution: Once the asynchronous operation is complete, the async function resumes execution with the result of the operation.
Async-Await Magic: Benefits of Async/Await:
- Improved Readability: Async/await makes your code look more like synchronous code, reducing the cognitive load on developers and improving code maintainability.
- Reduced Callback Hell: Eliminates nested callbacks, making your code more concise and easier to debug.
- Better Error Handling: Allows the use of familiar try/catch blocks for handling errors in asynchronous code, making error handling more intuitive.
- Simplified Concurrency: Makes it easier to write concurrent code, potentially improving application performance.
Async-Await Magic: Examples
Here is an example of how to use async/await to fetch data from an API:
async function getData() { const response = await fetch('https://api.example.com/data'); const data = await response.json(); return data; } getData().then(data => { console.log(data); });
This code is much easier to read and understand than the equivalent code using callbacks.
Conclusion
Async/await is a powerful tool that significantly simplifies asynchronous programming, making your code more readable, maintainable, and error-resistant. If you are not yet using async/await, give it a try. It might just be the magic spell you need to tame the asynchronous beast.
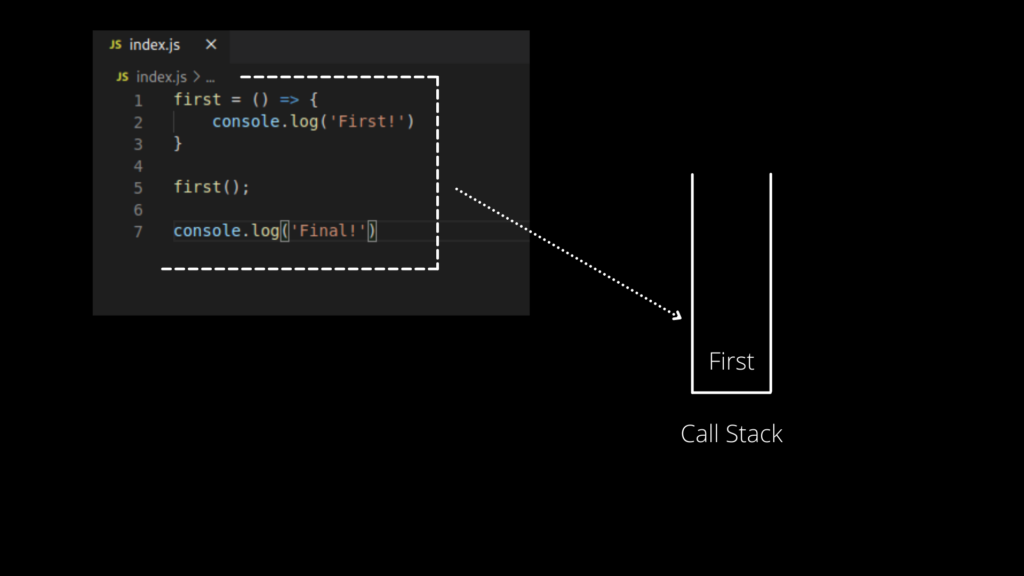
Additional Tips:
- Use try/catch blocks to handle errors in your async functions.
- Avoid nesting async functions too deeply to maintain code readability.
- Consider using async/await in conjunction with other asynchronous features like Promises and generators.
I hope this blog has helped you understand how async/await can simplify asynchronous programming.
JavaScript Simplicity: Seamless async/await and Promises for Best Readability – 5 Facts
Leave A Comment